Note
Go to the end to download the full example code.
Computing a simple NLTE 8542 line profile in a FAL C atmosphere
First, we import everything we need. Lightweaver is typically imported as lw, but things like the library of model atoms and Fal atmospheres need to be imported separately.
from lightweaver.fal import Falc82
from lightweaver.rh_atoms import H_6_atom, C_atom, O_atom, Si_atom, Al_atom, \
CaII_atom, Fe_atom, He_9_atom, MgII_atom, N_atom, Na_atom, S_atom
import lightweaver as lw
import matplotlib.pyplot as plt
import time
import numpy as np
Now, we define the functions that will be used in our spectral synthesise. First synth_8542 which synthesises and returns the line given by an atmosphere.
def synth_8542(atmos, conserve, useNe, wave):
'''
Synthesise a spectral line for given atmosphere with different
conditions.
Parameters
----------
atmos : lw.Atmosphere
The atmospheric model in which to synthesise the line.
conserve : bool
Whether to start from LTE electron density and conserve charge, or
simply use from the electron density present in the atomic model.
useNe : bool
Whether to use the electron density present in the model as the
starting solution, or compute the LTE electron density.
wave : np.ndarray
Array of wavelengths over which to resynthesise the final line
profile for muz=1.
Returns
-------
ctx : lw.Context
The Context object that was used to compute the equilibrium
populations.
Iwave : np.ndarray
The intensity at muz=1 for each wavelength in `wave`.
'''
# Configure the atmospheric angular quadrature
atmos.quadrature(5)
# Configure the set of atomic models to use.
aSet = lw.RadiativeSet([H_6_atom(), C_atom(), O_atom(), Si_atom(),
Al_atom(), CaII_atom(), Fe_atom(), He_9_atom(),
MgII_atom(), N_atom(), Na_atom(), S_atom()
])
# Set H and Ca to "active" i.e. NLTE, everything else participates as an
# LTE background.
aSet.set_active('H', 'Ca')
# Compute the necessary wavelength dependent information (SpectrumConfiguration).
spect = aSet.compute_wavelength_grid()
# Either compute the equilibrium populations at the fixed electron density
# provided in the model, or iterate an LTE electron density and compute the
# corresponding equilibrium populations (SpeciesStateTable).
if useNe:
eqPops = aSet.compute_eq_pops(atmos)
else:
eqPops = aSet.iterate_lte_ne_eq_pops(atmos)
# Configure the Context which holds the state of the simulation for the
# backend, and provides the python interface to the backend.
# Feel free to increase Nthreads to increase the number of threads the
# program will use.
ctx = lw.Context(atmos, spect, eqPops, conserveCharge=conserve, Nthreads=1)
# Iterate the Context to convergence (using the iteration function now
# provided by Lightweaver)
lw.iterate_ctx_se(ctx)
# Update the background populations based on the converged solution and
# compute the final intensity for mu=1 on the provided wavelength grid.
eqPops.update_lte_atoms_Hmin_pops(atmos)
Iwave = ctx.compute_rays(wave, [atmos.muz[-1]], stokes=False)
return ctx, Iwave
The wavelength grid to output the final synthesised line on.
wave = np.linspace(853.9444, 854.9444, 1001)
Load an lw.Atmosphere object containing the FAL C atmosphere with 82 points in depth, before synthesising the Ca II 8542 AA line profile using:
The given electron density.
The electron density charge conserved from a starting LTE solution.
The LTE electron density.
These results are then plotted.
atmosRef = Falc82()
ctxRef, IwaveRef = synth_8542(atmosRef, conserve=False, useNe=True, wave=wave)
atmosCons = Falc82()
ctxCons, IwaveCons = synth_8542(atmosCons, conserve=True, useNe=False, wave=wave)
atmosLte = Falc82()
ctx, IwaveLte = synth_8542(atmosLte, conserve=False, useNe=False, wave=wave)
plt.plot(wave, IwaveRef, label='Reference FAL')
plt.plot(wave, IwaveCons, label='Reference Cons')
plt.plot(wave, IwaveLte, label='Reference LTE n_e')
plt.legend()
plt.show()
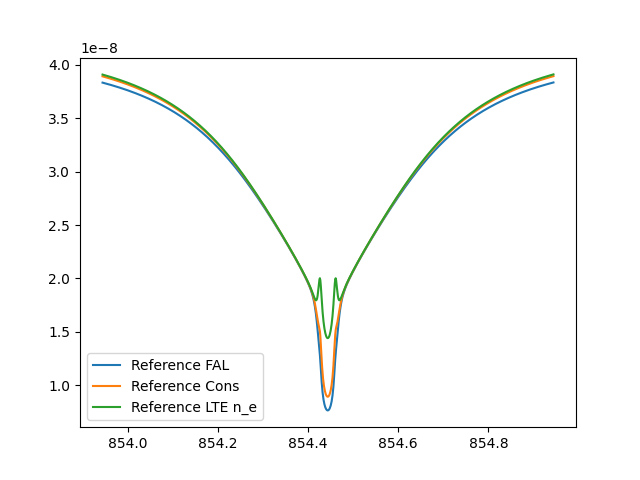
-- Iteration 0:
dJ = 1.00e+00
(Lambda iterating background)
-- Iteration 6:
dJ = 2.44e+00
H delta = 5.9862e-01
Ca delta = 2.2187e-01
-- Iteration 12:
dJ = 3.76e-01
H delta = 2.0284e-01
Ca delta = 1.5172e-01
-- Iteration 18:
dJ = 1.41e-01
H delta = 7.3603e-02
Ca delta = 6.8821e-02
-- Iteration 24:
dJ = 9.68e-02
H delta = 5.3146e-02
Ca delta = 4.1829e-02
-- Iteration 30:
dJ = 7.96e-02
H delta = 4.8067e-02
Ca delta = 2.9514e-02
-- Iteration 36:
dJ = 7.28e-02
H delta = 4.3635e-02
Ca delta = 2.3167e-02
-- Iteration 42:
dJ = 6.66e-02
H delta = 3.7502e-02
Ca delta = 1.8113e-02
-- Iteration 48:
dJ = 5.96e-02
H delta = 3.1502e-02
Ca delta = 1.3700e-02
-- Iteration 54:
dJ = 5.04e-02
H delta = 2.5356e-02
Ca delta = 9.7035e-03
-- Iteration 60:
dJ = 3.91e-02
H delta = 2.0761e-02
Ca delta = 6.4322e-03
-- Iteration 66:
dJ = 2.74e-02
H delta = 1.5181e-02
Ca delta = 4.0496e-03
-- Iteration 72:
dJ = 1.79e-02
H delta = 1.0098e-02
Ca delta = 2.3932e-03
-- Iteration 78:
dJ = 1.08e-02
H delta = 6.2078e-03
Ca delta = 1.3748e-03
-- Iteration 84:
dJ = 6.30e-03
H delta = 3.6453e-03
Ca delta = 7.7639e-04
-- Iteration 90:
dJ = 3.56e-03
H delta = 2.0733e-03
Ca delta = 4.3477e-04
-- Iteration 95:
dJ = 2.18e-03
H delta = 1.2688e-03
Ca delta = 2.6115e-04
--------------------------------------------------------------------------------
Final Iteration: 98
--------------------------------------------------------------------------------
dJ = 1.61e-03
H delta = 9.3709e-04
Ca delta = 1.9178e-04
--------------------------------------------------------------------------------
Context converged to statistical equilibrium in 98 iterations after 3.93 s.
--------------------------------------------------------------------------------
LTE Iterations 1 (-- slowest convergence)
-- Iteration 0:
dJ = 1.00e+00
(Lambda iterating background)
-- Iteration 5:
dJ = 4.11e+00
H delta = 8.9232e-01
Ca delta = 3.6326e-01
ne delta = 1.8491e-03
-- Iteration 10:
dJ = 5.02e-01
H delta = 3.3673e-01
Ca delta = 1.7078e-01
ne delta = 6.0604e-12
-- Iteration 15:
dJ = 2.08e-01
H delta = 1.6041e-01
Ca delta = 8.6198e-02
ne delta = 4.8051e-14
-- Iteration 20:
dJ = 1.24e-01
H delta = 7.6390e-02
Ca delta = 5.3760e-02
ne delta = 1.3040e-15
-- Iteration 25:
dJ = 9.49e-02
H delta = 5.8891e-02
Ca delta = 3.6819e-02
ne delta = 2.4895e-15
-- Iteration 30:
dJ = 8.17e-02
H delta = 5.0039e-02
Ca delta = 2.6951e-02
ne delta = 1.5311e-15
-- Iteration 35:
dJ = 7.39e-02
H delta = 4.6385e-02
Ca delta = 2.0503e-02
ne delta = 1.8967e-15
-- Iteration 40:
dJ = 6.85e-02
H delta = 4.1500e-02
Ca delta = 1.5740e-02
ne delta = 1.8967e-15
-- Iteration 45:
dJ = 6.39e-02
H delta = 3.8626e-02
Ca delta = 1.1758e-02
ne delta = 1.3610e-15
-- Iteration 50:
dJ = 5.87e-02
H delta = 3.6134e-02
Ca delta = 8.3598e-03
ne delta = 1.5411e-15
-- Iteration 55:
dJ = 5.26e-02
H delta = 3.2942e-02
Ca delta = 5.6797e-03
ne delta = -4.0635e-16
-- Iteration 60:
dJ = 4.55e-02
H delta = 2.9086e-02
Ca delta = 3.6816e-03
ne delta = 1.0836e-15
-- Iteration 65:
dJ = 3.73e-02
H delta = 2.4371e-02
Ca delta = 2.5699e-03
ne delta = 4.7419e-16
-- Iteration 70:
dJ = 2.97e-02
H delta = 1.9655e-02
Ca delta = 2.2399e-03
ne delta = 1.7012e-15
-- Iteration 75:
dJ = 2.27e-02
H delta = 1.5180e-02
Ca delta = 1.8643e-03
ne delta = 0.0000e+00
-- Iteration 80:
dJ = 1.67e-02
H delta = 1.1227e-02
Ca delta = 1.4503e-03
ne delta = 1.0207e-15
-- Iteration 85:
dJ = 1.19e-02
H delta = 8.0264e-03
Ca delta = 1.0661e-03
ne delta = 1.5042e-15
-- Iteration 90:
dJ = 8.19e-03
H delta = 5.5474e-03
Ca delta = 7.5131e-04
ne delta = 1.1908e-15
-- Iteration 95:
dJ = 5.54e-03
H delta = 3.7581e-03
Ca delta = 5.1807e-04
ne delta = 1.1908e-15
-- Iteration 100:
dJ = 3.70e-03
H delta = 2.5138e-03
Ca delta = 3.5078e-04
ne delta = 1.7012e-15
-- Iteration 105:
dJ = 2.45e-03
H delta = 1.6689e-03
Ca delta = 2.3436e-04
ne delta = 9.4837e-16
-- Iteration 110:
dJ = 1.62e-03
H delta = 1.1013e-03
Ca delta = 1.5532e-04
ne delta = 7.0404e-16
--------------------------------------------------------------------------------
Final Iteration: 112
--------------------------------------------------------------------------------
dJ = 1.37e-03
H delta = 9.3152e-04
Ca delta = 1.3153e-04
ne delta = 1.5311e-15
--------------------------------------------------------------------------------
Context converged to statistical equilibrium in 112 iterations after 4.68 s.
--------------------------------------------------------------------------------
LTE Iterations 1 (-- slowest convergence)
-- Iteration 0:
dJ = 1.00e+00
(Lambda iterating background)
-- Iteration 6:
dJ = 1.69e+00
H delta = 5.9317e-01
Ca delta = 2.6750e-01
-- Iteration 12:
dJ = 2.89e-01
H delta = 1.4959e-01
Ca delta = 1.0630e-01
-- Iteration 18:
dJ = 1.32e-01
H delta = 7.3022e-02
Ca delta = 4.7099e-02
-- Iteration 24:
dJ = 9.42e-02
H delta = 5.5032e-02
Ca delta = 3.0148e-02
-- Iteration 30:
dJ = 7.69e-02
H delta = 4.9518e-02
Ca delta = 2.2515e-02
-- Iteration 36:
dJ = 6.80e-02
H delta = 4.4035e-02
Ca delta = 1.7365e-02
-- Iteration 42:
dJ = 6.06e-02
H delta = 3.6520e-02
Ca delta = 1.3000e-02
-- Iteration 48:
dJ = 5.17e-02
H delta = 2.7822e-02
Ca delta = 9.1776e-03
-- Iteration 54:
dJ = 4.09e-02
H delta = 2.1105e-02
Ca delta = 6.0669e-03
-- Iteration 60:
dJ = 2.87e-02
H delta = 1.5378e-02
Ca delta = 3.7084e-03
-- Iteration 66:
dJ = 1.86e-02
H delta = 1.0214e-02
Ca delta = 2.2082e-03
-- Iteration 72:
dJ = 1.15e-02
H delta = 6.3636e-03
Ca delta = 1.2646e-03
-- Iteration 78:
dJ = 6.58e-03
H delta = 3.6913e-03
Ca delta = 7.0107e-04
-- Iteration 84:
dJ = 3.70e-03
H delta = 2.0806e-03
Ca delta = 3.8579e-04
-- Iteration 90:
dJ = 2.04e-03
H delta = 1.1533e-03
Ca delta = 2.1069e-04
--------------------------------------------------------------------------------
Final Iteration: 92
--------------------------------------------------------------------------------
dJ = 1.67e-03
H delta = 9.4479e-04
Ca delta = 1.7206e-04
--------------------------------------------------------------------------------
Context converged to statistical equilibrium in 92 iterations after 3.66 s.
--------------------------------------------------------------------------------
LTE Iterations 1 (He slowest convergence)
Total running time of the script: (0 minutes 13.183 seconds)